Install
Add Dependency on adcio_placement (opens in a new tab)
dependencies:
adcio_placement: ^version
Initialize ADCIO Plugin
All plugins in the ADCIO receive the required ID through the adcio_core plugin.
Initialize using the initializeApp()
function in the main()
method to run only once at the beginning of the runtime.
AdcioCore.initializeApp(clientId = "YOUR_ADCIO_CLIENT_ID");
For information on how to get the clientId, please refer here
Making a Suggestion
You can get recommendations from ADCIO via the adcioCreateSuggestion function. You can call the function with only placementId in the parameters, but if you have additional information such as customerId (userId), age, gender, area, placementPosition, the recommendation prediction accuracy will be higher.
For more detailed information about suggestions, please refer to the documentation.
import 'package:adcio_placement/adcio_placement.dart';
adcioCreateSuggestion(
placementId: '...',
).then((result) {
AdcioSuggestionRawData adcioSuggestionRaw = result;
...
// The 'idOnStore' value matches the product ID in the client development service
final productId = adcioSuggestionRaw.suggestions[i].product.idOnStore;
});
The adcioCreateSuggestion function returns an Future<AdcioSuggestionRawData>
object.
Parameters
Please refer to the Recommendations API documentation
property | description | type | default |
---|---|---|---|
placementId | Your Placement Id | String | required |
customerId | Customer Id | String? | null |
placementPosition | placement position offset | Offest? | null |
birthYear | customer birth year | int? | null |
gender | customer gender | String? | null |
area | customer area | String? | null |
Response
Please refer to the Recommendations API documentation
Example Full Code
Preview Image
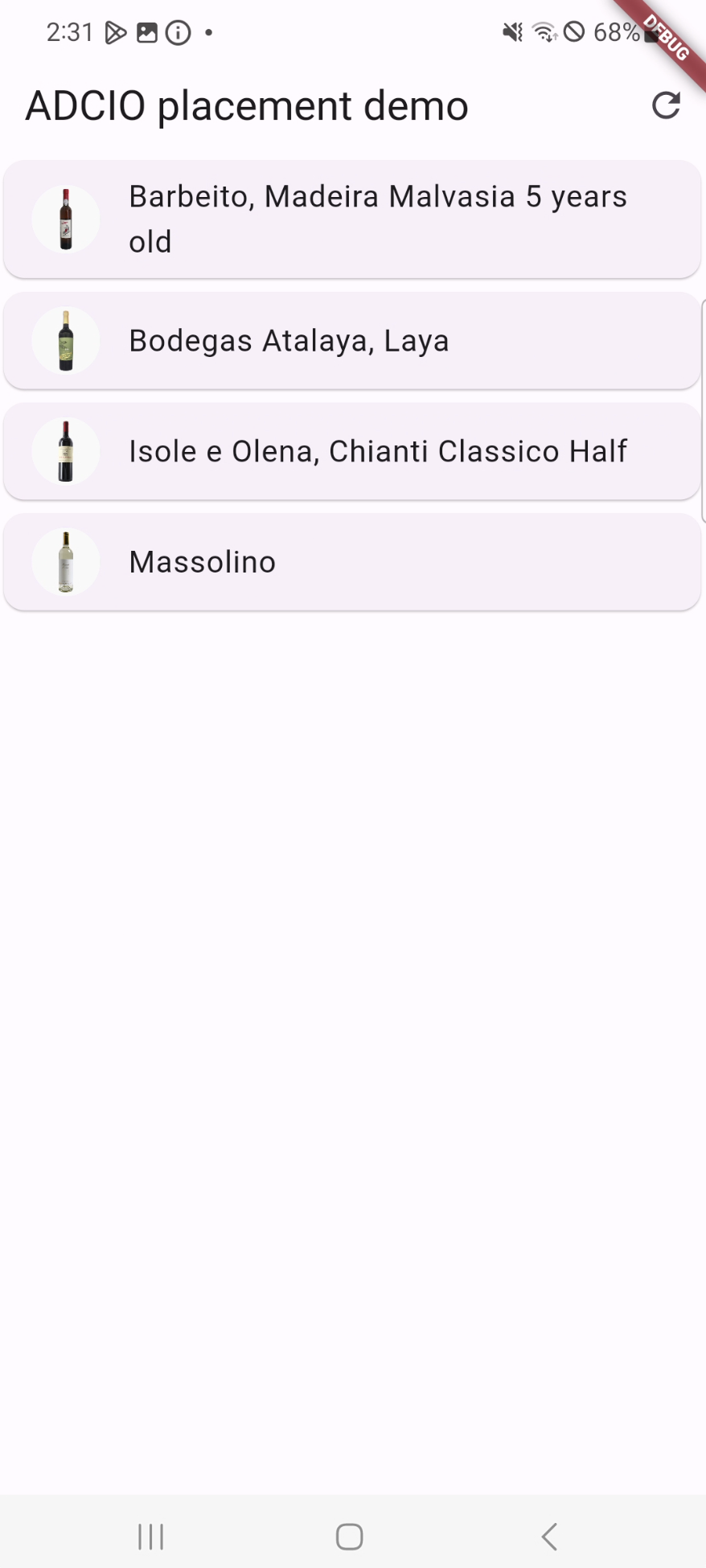
Code
example/lib/main.dart (opens in a new tab)
// ignore_for_file: use_build_context_synchronously
import 'package:adcio_core/adcio_core.dart';
import 'package:adcio_placement/adcio_placement.dart';
import 'package:example/model/user.dart';
import 'package:flutter/material.dart';
import 'package:uuid/uuid.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
/// this is important to call `AdcioCore.initializeApp(clientId: 'ADCIO_STORE_ID')` function.
await AdcioCore.initializeApp(
clientId: 'f8f2e298-c168-4412-b82d-98fc5b4a114a',
);
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
AdcioSuggestionRawData? rawData;
late final User currenctUser;
late final String currentLocation;
@override
void initState() {
super.initState();
currenctUser = User(
id: const Uuid().v4(),
name: 'adcio',
birthDate: DateTime(2000, 2, 20),
gender: Gender.male,
);
currentLocation = 'Seoul, Korea';
suggest();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ADCIO placement demo'),
actions: [
IconButton(
onPressed: () {
suggest();
},
icon: const Icon(Icons.refresh),
),
],
),
body: rawData == null
? const Center(child: CircularProgressIndicator())
: ListView.builder(
itemBuilder: (context, index) => Card(
///
/// used suggestion data
child: ListTile(
onTap: () => debugPrint(
'Product ID for your service: ${rawData!.suggestions[index].product!.idOnStore}',
),
leading: CircleAvatar(
backgroundImage: NetworkImage(
rawData!.suggestions[index].product!.image,
),
),
title: Text(
rawData!.suggestions[index].product!.name,
),
),
),
itemCount: rawData!.suggestions.length,
),
);
}
///
/// call adcioSuggest() here
void suggest() {
adcioCreateSuggestion(
placementId: '67592c00-a230-4c31-902e-82ae4fe71866',
customerId: currenctUser.id,
birthYear: currenctUser.birthDate.year,
gender: currenctUser.gender.name,
area: currentLocation,
).then((value) {
rawData = value;
setState(() {});
});
}
}